diff --git a/.gitignore b/.gitignore
new file mode 100644
index 0000000..07b0a62
--- /dev/null
+++ b/.gitignore
@@ -0,0 +1,6 @@
+# Project exclude paths
+/domain/target/
+/email-app/target/
+/repository/target/
+/service/target/
+/web/target/
\ No newline at end of file
diff --git a/README.md b/README.md
index aee569d..56dfa26 100644
--- a/README.md
+++ b/README.md
@@ -1,6 +1,13 @@
# spring-multi-module-application
How to create Maven multi module project in spring boot
+To package and run it, just execute:
+```console
+mvn clean package
+cd web/target
+java -jar web-0.0.1-SNAPSHOT.jar
+```
+
# project structure
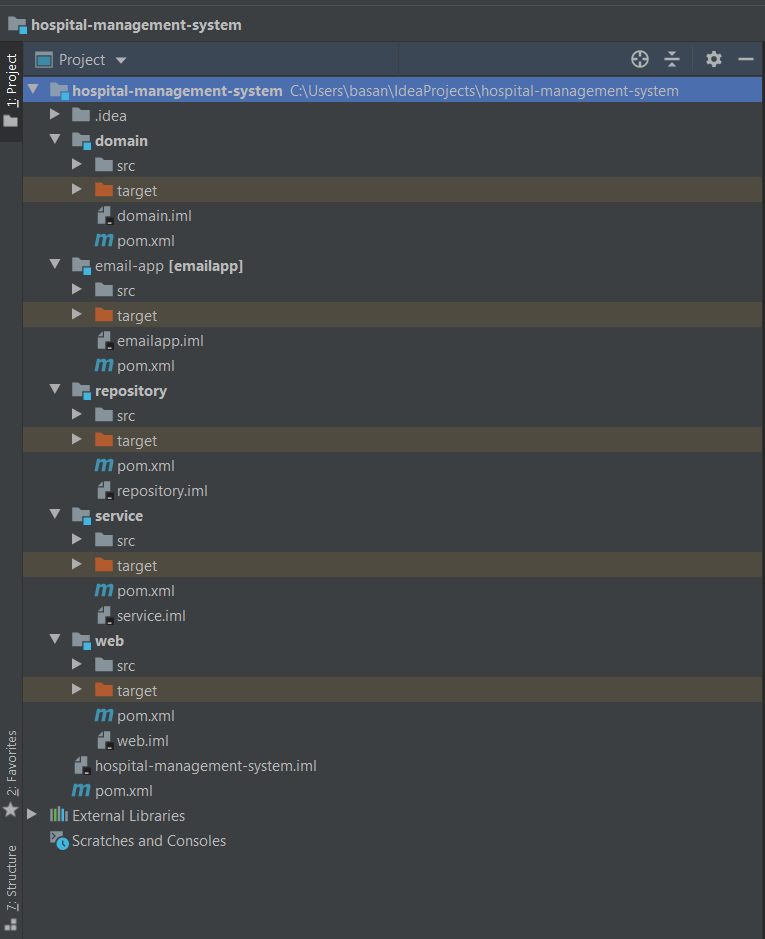
diff --git a/domain/pom.xml b/domain/pom.xml
index a489877..ec28528 100644
--- a/domain/pom.xml
+++ b/domain/pom.xml
@@ -10,6 +10,6 @@
4.0.0
domain
-jar
+ jar
\ No newline at end of file
diff --git a/domain/src/main/java/com/javatechie/model/doctor/Doctor.java b/domain/src/main/java/com/javatechie/model/doctor/Doctor.java
index 63ca8c4..3a2ad31 100644
--- a/domain/src/main/java/com/javatechie/model/doctor/Doctor.java
+++ b/domain/src/main/java/com/javatechie/model/doctor/Doctor.java
@@ -1,21 +1,45 @@
package com.javatechie.model.doctor;
-import lombok.AllArgsConstructor;
-import lombok.Data;
-import lombok.NoArgsConstructor;
-import lombok.ToString;
-
import javax.persistence.Entity;
import javax.persistence.Id;
-@Data
-@AllArgsConstructor
-@NoArgsConstructor
-@ToString
@Entity
public class Doctor {
@Id
private int id;
private String name;
private String specialist;
+
+ public Doctor() {
+ }
+
+ public Doctor(int id, String name, String specialist) {
+ this.id = id;
+ this.name = name;
+ this.specialist = specialist;
+ }
+
+ public int getId() {
+ return id;
+ }
+
+ public void setId(int id) {
+ this.id = id;
+ }
+
+ public String getName() {
+ return name;
+ }
+
+ public void setName(String name) {
+ this.name = name;
+ }
+
+ public String getSpecialist() {
+ return specialist;
+ }
+
+ public void setSpecialist(String specialist) {
+ this.specialist = specialist;
+ }
}
diff --git a/hospital-management-system.iml b/hospital-management-system.iml
new file mode 100644
index 0000000..037adc5
--- /dev/null
+++ b/hospital-management-system.iml
@@ -0,0 +1,78 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/pom.xml b/pom.xml
index 165aa7c..3289312 100644
--- a/pom.xml
+++ b/pom.xml
@@ -8,13 +8,8 @@
service
repository
web
- email
- email
- email
- email
- email-api
- email
email-app
+
org.springframework.boot
@@ -30,8 +25,10 @@
1.8
+ main.HMSApplication
+
org.springframework.boot
@@ -44,11 +41,7 @@
h2
runtime
-
- org.projectlombok
- lombok
- true
-
+
org.springframework.boot
spring-boot-starter-test
@@ -56,13 +49,4 @@
-
-
-
- org.springframework.boot
- spring-boot-maven-plugin
-
-
-
-
diff --git a/service/service.iml b/service/service.iml
new file mode 100644
index 0000000..8c83624
--- /dev/null
+++ b/service/service.iml
@@ -0,0 +1,82 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/service/src/main/java/com/javatechie/service/doctor/DoctorService.java b/service/src/main/java/com/javatechie/service/doctor/DoctorService.java
index 240a06d..fd3d041 100644
--- a/service/src/main/java/com/javatechie/service/doctor/DoctorService.java
+++ b/service/src/main/java/com/javatechie/service/doctor/DoctorService.java
@@ -5,7 +5,6 @@
import com.javatechie.model.doctor.Doctor;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
-
import javax.annotation.PostConstruct;
import java.util.List;
import java.util.stream.Collectors;
diff --git a/web/pom.xml b/web/pom.xml
index 87d9a03..a1919dd 100644
--- a/web/pom.xml
+++ b/web/pom.xml
@@ -12,6 +12,7 @@
web
jar
+
com.javatechie
@@ -23,4 +24,13 @@
spring-boot-starter-web
+
+
+
+
+ org.springframework.boot
+ spring-boot-maven-plugin
+
+
+
\ No newline at end of file
diff --git a/web/src/main/java/main/HMSApplication.java b/web/src/main/java/main/HMSApplication.java
new file mode 100644
index 0000000..56994b3
--- /dev/null
+++ b/web/src/main/java/main/HMSApplication.java
@@ -0,0 +1,19 @@
+package main;
+
+import org.springframework.boot.SpringApplication;
+import org.springframework.boot.autoconfigure.SpringBootApplication;
+import org.springframework.boot.autoconfigure.domain.EntityScan;
+import org.springframework.context.annotation.ComponentScan;
+import org.springframework.data.jpa.repository.config.EnableJpaRepositories;
+
+@SpringBootApplication
+@ComponentScan(basePackages = {"com.javatechie.*"})
+@EntityScan(basePackages = {"com.javatechie.*"})
+@EnableJpaRepositories(basePackages = {"com.javatechie.*"})
+public class HMSApplication {
+
+ public static void main(String[] args) {
+
+ SpringApplication.run(HMSApplication.class);
+ }
+}