Commit e0f2ff2
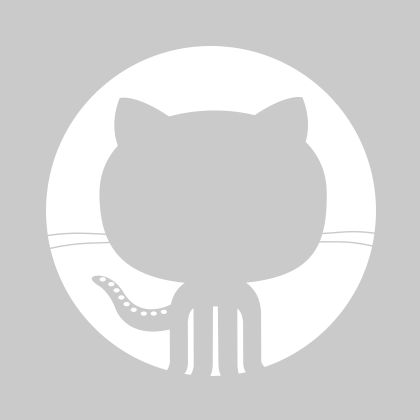
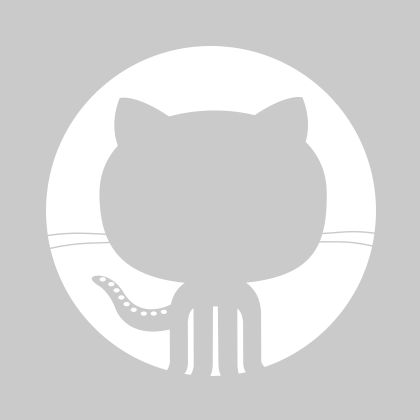
Preslav Le
Convex, Inc.
1 parent aa99e12 commit e0f2ff2
File tree
6 files changed
+97
-48
lines changed- crates
- common/src
- http
- local_backend/src
- sync/src
6 files changed
+97
-48
lines changedDiff for: crates/common/src/execution_context.rs
+4
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
100 | 100 |
| |
101 | 101 |
| |
102 | 102 |
| |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
103 | 107 |
| |
104 | 108 |
| |
105 | 109 |
| |
|
Diff for: crates/common/src/http/mod.rs
+62-3
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
8 |
| - | |
9 |
| - | |
10 |
| - | |
| 8 | + | |
11 | 9 |
| |
12 | 10 |
| |
13 | 11 |
| |
| |||
70 | 68 |
| |
71 | 69 |
| |
72 | 70 |
| |
| 71 | + | |
73 | 72 |
| |
74 | 73 |
| |
75 | 74 |
| |
| |||
94 | 93 |
| |
95 | 94 |
| |
96 | 95 |
| |
| 96 | + | |
97 | 97 |
| |
98 | 98 |
| |
99 | 99 |
| |
100 | 100 |
| |
101 | 101 |
| |
| 102 | + | |
102 | 103 |
| |
103 | 104 |
| |
104 | 105 |
| |
| |||
707 | 708 |
| |
708 | 709 |
| |
709 | 710 |
| |
| 711 | + | |
710 | 712 |
| |
711 | 713 |
| |
712 | 714 |
| |
| |||
725 | 727 |
| |
726 | 728 |
| |
727 | 729 |
| |
| 730 | + | |
| 731 | + | |
| 732 | + | |
| 733 | + | |
| 734 | + | |
| 735 | + | |
| 736 | + | |
| 737 | + | |
| 738 | + | |
| 739 | + | |
| 740 | + | |
| 741 | + | |
728 | 742 |
| |
729 | 743 |
| |
730 | 744 |
| |
| |||
804 | 818 |
| |
805 | 819 |
| |
806 | 820 |
| |
| 821 | + | |
| 822 | + | |
| 823 | + | |
| 824 | + | |
| 825 | + | |
| 826 | + | |
| 827 | + | |
| 828 | + | |
| 829 | + | |
| 830 | + | |
| 831 | + | |
| 832 | + | |
| 833 | + | |
| 834 | + | |
| 835 | + | |
| 836 | + | |
| 837 | + | |
| 838 | + | |
| 839 | + | |
| 840 | + | |
| 841 | + | |
| 842 | + | |
| 843 | + | |
| 844 | + | |
| 845 | + | |
| 846 | + | |
| 847 | + | |
| 848 | + | |
| 849 | + | |
| 850 | + | |
| 851 | + | |
| 852 | + | |
| 853 | + | |
| 854 | + | |
| 855 | + | |
| 856 | + | |
| 857 | + | |
| 858 | + | |
| 859 | + | |
| 860 | + | |
| 861 | + | |
| 862 | + | |
| 863 | + | |
| 864 | + | |
| 865 | + | |
807 | 866 |
| |
808 | 867 |
| |
809 | 868 |
| |
|
Diff for: crates/common/src/minitrace_helpers.rs
+9-11
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 |
| - | |
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
2 | 5 |
| |
3 | 6 |
| |
4 | 7 |
| |
5 | 8 |
| |
6 | 9 |
| |
7 | 10 |
| |
8 | 11 |
| |
9 |
| - | |
10 |
| - | |
11 |
| - | |
12 |
| - | |
| 12 | + | |
13 | 13 |
| |
14 | 14 |
| |
15 | 15 |
| |
| |||
27 | 27 |
| |
28 | 28 |
| |
29 | 29 |
| |
30 |
| - | |
31 |
| - | |
32 |
| - | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
33 | 33 |
| |
34 | 34 |
| |
35 |
| - | |
36 |
| - | |
37 |
| - | |
| 35 | + | |
38 | 36 |
| |
39 | 37 |
| |
40 | 38 |
| |
|
+5-6
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
17 | 17 |
| |
18 | 18 |
| |
19 | 19 |
| |
20 |
| - | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
21 | 24 |
| |
22 |
| - | |
23 | 25 |
| |
24 | 26 |
| |
25 | 27 |
| |
| |||
87 | 89 |
| |
88 | 90 |
| |
89 | 91 |
| |
| 92 | + | |
90 | 93 |
| |
91 | 94 |
| |
92 | 95 |
| |
| |||
97 | 100 |
| |
98 | 101 |
| |
99 | 102 |
| |
100 |
| - | |
101 |
| - | |
102 |
| - | |
103 |
| - | |
104 | 103 |
| |
105 | 104 |
| |
106 | 105 |
| |
|
Diff for: crates/local_backend/src/public_api.rs
+7-20
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
17 | 17 |
| |
18 | 18 |
| |
19 | 19 |
| |
| 20 | + | |
20 | 21 |
| |
21 | 22 |
| |
22 | 23 |
| |
| |||
25 | 26 |
| |
26 | 27 |
| |
27 | 28 |
| |
28 |
| - | |
29 | 29 |
| |
30 | 30 |
| |
31 | 31 |
| |
| |||
137 | 137 |
| |
138 | 138 |
| |
139 | 139 |
| |
| 140 | + | |
140 | 141 |
| |
141 | 142 |
| |
142 | 143 |
| |
| |||
147 | 148 |
| |
148 | 149 |
| |
149 | 150 |
| |
150 |
| - | |
151 |
| - | |
152 |
| - | |
153 |
| - | |
154 | 151 |
| |
155 | 152 |
| |
156 | 153 |
| |
| |||
205 | 202 |
| |
206 | 203 |
| |
207 | 204 |
| |
| 205 | + | |
208 | 206 |
| |
209 | 207 |
| |
210 | 208 |
| |
211 | 209 |
| |
212 | 210 |
| |
213 | 211 |
| |
214 | 212 |
| |
215 |
| - | |
216 |
| - | |
217 |
| - | |
218 | 213 |
| |
219 | 214 |
| |
220 | 215 |
| |
| |||
242 | 237 |
| |
243 | 238 |
| |
244 | 239 |
| |
| 240 | + | |
245 | 241 |
| |
246 | 242 |
| |
247 | 243 |
| |
| |||
250 | 246 |
| |
251 | 247 |
| |
252 | 248 |
| |
253 |
| - | |
254 |
| - | |
255 |
| - | |
256 | 249 |
| |
257 | 250 |
| |
258 | 251 |
| |
| |||
289 | 282 |
| |
290 | 283 |
| |
291 | 284 |
| |
| 285 | + | |
292 | 286 |
| |
293 | 287 |
| |
294 | 288 |
| |
295 | 289 |
| |
296 | 290 |
| |
297 | 291 |
| |
298 |
| - | |
299 |
| - | |
300 |
| - | |
301 | 292 |
| |
302 | 293 |
| |
303 | 294 |
| |
| |||
333 | 324 |
| |
334 | 325 |
| |
335 | 326 |
| |
| 327 | + | |
336 | 328 |
| |
337 | 329 |
| |
338 | 330 |
| |
339 | 331 |
| |
340 | 332 |
| |
341 |
| - | |
342 |
| - | |
343 |
| - | |
344 | 333 |
| |
345 | 334 |
| |
346 | 335 |
| |
| |||
372 | 361 |
| |
373 | 362 |
| |
374 | 363 |
| |
| 364 | + | |
375 | 365 |
| |
376 | 366 |
| |
377 | 367 |
| |
378 | 368 |
| |
379 |
| - | |
380 |
| - | |
381 |
| - | |
382 | 369 |
| |
383 | 370 |
| |
384 | 371 |
| |
|
Diff for: crates/sync/src/worker.rs
+10-8
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
430 | 430 |
| |
431 | 431 |
| |
432 | 432 |
| |
433 |
| - | |
434 |
| - | |
435 |
| - | |
436 |
| - | |
437 |
| - | |
438 |
| - | |
439 |
| - | |
440 |
| - | |
| 433 | + | |
| 434 | + | |
| 435 | + | |
| 436 | + | |
| 437 | + | |
| 438 | + | |
| 439 | + | |
| 440 | + | |
| 441 | + | |
| 442 | + | |
441 | 443 |
| |
442 | 444 |
| |
443 | 445 |
| |
|
0 commit comments