Commit 2c32e71
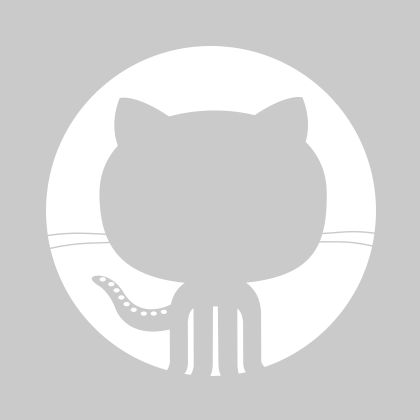
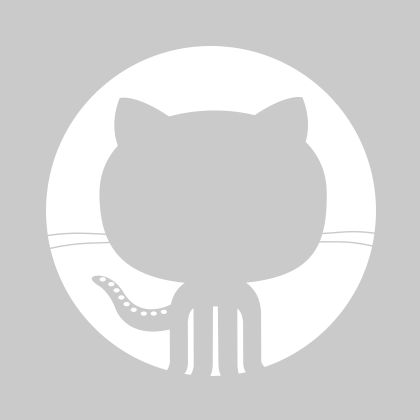
Jonathan Kliem
Matthias Koeppe
1 parent d441cbf commit 2c32e71
File tree
5 files changed
+127
-1
lines changed- docs/source
- src/cysignals
5 files changed
+127
-1
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
47 | 47 |
| |
48 | 48 |
| |
49 | 49 |
| |
| 50 | + | |
50 | 51 |
| |
51 | 52 |
| |
52 | 53 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
249 | 249 |
| |
250 | 250 |
| |
251 | 251 |
| |
| 252 | + | |
| 253 | + | |
| 254 | + | |
| 255 | + | |
| 256 | + | |
| 257 | + | |
| 258 | + | |
| 259 | + | |
| 260 | + | |
| 261 | + | |
| 262 | + | |
| 263 | + | |
| 264 | + | |
| 265 | + | |
| 266 | + | |
| 267 | + | |
| 268 | + | |
| 269 | + | |
| 270 | + | |
| 271 | + | |
| 272 | + | |
| 273 | + | |
| 274 | + | |
| 275 | + | |
| 276 | + | |
| 277 | + | |
| 278 | + | |
| 279 | + | |
| 280 | + | |
| 281 | + | |
| 282 | + | |
| 283 | + | |
| 284 | + | |
| 285 | + | |
| 286 | + | |
| 287 | + | |
| 288 | + | |
| 289 | + | |
| 290 | + | |
| 291 | + | |
| 292 | + | |
| 293 | + | |
| 294 | + | |
| 295 | + | |
| 296 | + | |
| 297 | + | |
| 298 | + | |
| 299 | + | |
| 300 | + | |
| 301 | + | |
| 302 | + | |
| 303 | + | |
| 304 | + | |
| 305 | + | |
| 306 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
62 | 62 |
| |
63 | 63 |
| |
64 | 64 |
| |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
65 | 96 |
| |
66 | 97 |
| |
67 | 98 |
| |
| |||
214 | 245 |
| |
215 | 246 |
| |
216 | 247 |
| |
217 |
| - | |
| 248 | + | |
218 | 249 |
| |
219 | 250 |
| |
220 | 251 |
| |
| |||
238 | 269 |
| |
239 | 270 |
| |
240 | 271 |
| |
| 272 | + | |
241 | 273 |
| |
242 | 274 |
| |
243 | 275 |
| |
| |||
400 | 432 |
| |
401 | 433 |
| |
402 | 434 |
| |
| 435 | + | |
403 | 436 |
| |
404 | 437 |
| |
405 | 438 |
| |
| |||
412 | 445 |
| |
413 | 446 |
| |
414 | 447 |
| |
| 448 | + | |
415 | 449 |
| |
416 | 450 |
| |
417 | 451 |
| |
| 452 | + | |
418 | 453 |
| |
419 | 454 |
| |
420 | 455 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
49 | 49 |
| |
50 | 50 |
| |
51 | 51 |
| |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
52 | 56 |
| |
53 | 57 |
| |
54 | 58 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
54 | 54 |
| |
55 | 55 |
| |
56 | 56 |
| |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
57 | 63 |
| |
58 | 64 |
| |
59 | 65 |
| |
| |||
74 | 80 |
| |
75 | 81 |
| |
76 | 82 |
| |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
77 | 108 |
| |
78 | 109 |
| |
79 | 110 |
| |
|
0 commit comments